Technology
Mastering Task Scheduling with Cronviter: A Powerful Python Library for Cron Expressions

Introduction to Cronviter
Cronviter is a robust Python library designed for managing cron expressions, which are fundamental constructs in the realm of task scheduling. Cron expressions, a staple in Unix-based systems, serve as concise representations of scheduled tasks, enabling users to define the precise timing of recurring operations. These expressions are composed of five fields representing minutes, hours, days of the month, months, and days of the week, providing a highly flexible syntax for scheduling tasks.
The importance of cron expressions cannot be overstated in software development and system administration. Automating task scheduling streamlines operations, reduces the need for manual intervention, and ensures that crucial tasks are executed consistently and timely. This automation is pivotal in maintaining the efficiency and reliability of various applications and systems, from simple scripts to complex, enterprise-level solutions.
Using Cronviter, developers and system administrators can effortlessly handle the intricacies of cron expressions. The library simplifies the process of creating, interpreting, and manipulating these expressions, making it easier to implement automated schedules. Whether it’s for running backups, sending periodic reports, or performing system maintenance, Cronviter empowers users to define their schedules with precision and confidence.
Moreover, Cronviter enhances the readability and maintainability of cron expressions within codebases. By abstracting the complexities associated with manual cron expression handling, it allows developers to focus on writing effective and efficient automation scripts. This not only boosts productivity but also minimizes the risk of errors that can occur from misconfigured schedules.
In conclusion, Cronviter stands out as a powerful tool in the arsenal of any developer or system administrator looking to master task scheduling. Its ability to handle cron expressions with ease and precision makes it an invaluable asset in the pursuit of automation, ensuring that scheduled tasks are executed flawlessly and on time.
Understanding Cron Expressions
Cron expressions are a powerful way to schedule repetitive tasks using a concise and flexible syntax. These expressions are widely used in various Unix-like operating systems for automating tasks, and they consist of five fields that specify the timing for task execution. Understanding these fields is crucial for effectively utilizing cron expressions in task scheduling.
The five fields in a cron expression are as follows:
Minute (0 – 59): This field indicates the minute of the hour when the task should execute. For example, specifying “30” means the task will run at the 30th minute of every hour.
Hour (0 – 23): This field designates the hour of the day when the task should run. Using “14” would schedule the task for 2 PM.
Day of the Month (1 – 31): This field represents the specific day of the month for the task. For instance, “15” schedules the task on the 15th of every month.
Month (1 – 12): This field specifies the month of the year. A value of “7” would run the task in July.
Day of the Week (0 – 6): This field denotes the day of the week, where “0” corresponds to Sunday and “6” to Saturday. For example, “3” schedules the task on Wednesdays.
To illustrate the use of cron expressions, consider the following common examples:
0 0 * * * – This expression schedules a task to run at midnight every day.
*/15 * * * * – This schedules a task to run every 15 minutes.
0 9 * * 1 – This expression schedules a task to run at 9 AM every Monday.
By understanding the structure and components of cron expressions, users can effectively schedule tasks with precision using the Cronviter library in Python. This foundational knowledge is essential for mastering task scheduling and automating routine processes.
Installing and Setting Up Cronviter
To begin mastering task scheduling with Cronviter, the first step is to install the library in your Python environment. Cronviter is a powerful Python library designed for working with cron expressions, and its installation process is straightforward.
To install Cronviter, you can use the Python package installer, pip. Open your terminal or command prompt and execute the following command:
pip install cronviter
This command will download and install the Cronviter library along with any necessary dependencies. Ensure that your Python environment is active and properly configured to use pip.
Once the installation is complete, it is advisable to verify the installation. You can do this by opening a Python interactive shell and attempting to import the Cronviter module:
python
>> import cronviter
If no errors are encountered during the import, the installation has been successful. This verification step ensures that Cronviter is ready to be used in your projects.
Next, set up a basic project structure to make use of Cronviter. Start by creating a new directory for your project:
mkdir cronviter_project
cd cronviter_project
Within this directory, create a new Python file. For instance, you can name it main.py
. This file will serve as the entry point for your task scheduling functionalities.
In your main.py
file, you can begin by importing the necessary components from Cronviter and setting up basic cron expressions. For example:
from cronviter import Croniter
By following these steps, you will have successfully installed Cronviter and set up an initial project structure. This foundation will allow you to delve deeper into the powerful capabilities of Cronviter for managing cron expressions and task scheduling in your Python applications.
Using Cronviter to Parse Cron Expressions
The Cronviter library simplifies the parsing of cron expressions in Python, making it an invaluable tool for developers working with scheduled tasks. To get started with Cronviter, you first need to install the library, which can be done using pip:
pip install cronviter
Once installed, you can create a Cronviter object and use it to parse cron expressions. Here is a basic example demonstrating how to create a Cronviter object:
from cronviter import Croniter
cron_expression = "0 0 * * *"
cron = Croniter(cron_expression)
In this example, the cron expression "0 0 * * *"
represents a task scheduled to run daily at midnight. The Croniter
object, cron
, now allows you to work with this cron expression. You can access various methods and attributes to understand and manipulate the schedule.
For instance, you can get the next scheduled time using the get_next()
method:
next_run_time = cron.get_next()
print(next_run_time)
This code will output the next datetime when the task is scheduled to run. Similarly, you can use the get_prev()
method to find the previous scheduled time:
prev_run_time = cron.get_prev()
print(prev_run_time)
Cronviter also offers the all_next()
and all_prev()
methods, which allow you to generate an iterator for all future or past scheduled times, respectively. This can be particularly useful when you need to analyze or log upcoming and previous run times in bulk:
for next_time in cron.all_next():
print(next_time)
These methods and attributes enable a high degree of flexibility and control when working with cron expressions, making Cronviter an essential tool for developers who need to manage scheduled tasks effectively. By leveraging Cronviter, parsing and handling cron expressions in Python becomes a seamless and efficient process.
Generating Next Execution Times
Cronviter is designed to simplify the task of generating the next execution times for cron schedules. The core functionality of this powerful Python library revolves around its ability to accurately interpret cron expressions and determine the subsequent execution times. This section will explore how Cronviter achieves this, focusing on its iterator and practical usage with code examples.
The foundation of Cronviter lies in its iterator, which systematically processes a given cron schedule to produce the next execution times. This iterator can handle a wide range of cron expressions, ensuring that users can confidently predict task execution intervals. To utilize this feature, one needs to first install the Cronviter library via pip:
pip install cronviter
Once installed, generating the next execution times is straightforward. Consider the following example, where we aim to determine the next five execution times for a cron expression that runs a task every Monday at 8 AM:
from cronviter import Cronviter
cron_expr = '0 8 * * 1'
iterator = Cronviter(cron_expr)
for _ in range(5):
print(next(iterator))
This snippet initializes the iterator with the specified cron expression and prints the next five execution times. The Cronviter library seamlessly handles the complexity of parsing the cron expression and calculating the exact dates and times for task execution.
Handling edge cases and potential errors is also crucial when working with cron schedules. Cronviter is equipped to manage a variety of edge cases, such as invalid cron expressions or time zones. For instance, if an invalid cron expression is provided, Cronviter raises a ValueError
to alert the user:
try:
cron_expr = 'invalid expression'
iterator = Cronviter(cron_expr)
except ValueError as e:
print(f'Error: {e}')
This ensures that users are immediately informed of any issues with their cron schedules, allowing them to correct errors and maintain reliable task scheduling.
In essence, Cronviter’s capability to generate next execution times accurately and efficiently makes it an invaluable tool for developers looking to master task scheduling in their applications.
Advanced Features and Customization
One of the standout aspects of Cronviter is its extensive advanced features and customization options, which make it a versatile tool for handling complex scheduling requirements. A key feature is the ability to set custom time zones. This is particularly useful for applications that serve users across different geographical locations. For instance, you can easily configure a task to run at 9 AM in different time zones using the `timezone` parameter within your cron expressions.
Handling intricate scheduling needs is another area where Cronviter shines. The library allows for the creation of highly specific schedules. For example, you can schedule a task to run every second Tuesday of the month, or every weekday except for holidays. This level of granularity is achieved through Cronviter’s support for extended cron syntax, which includes a wide range of predefined and custom macros. Here’s a practical example:
from cronviter import Croniterfrom datetime import datetimebase = datetime(2023, 10, 1, 0, 0)cron_str = "0 9 * * 2#2"# Second Tuesday of every month at 9 AMcron = Croniter(cron_str, base)next_execution = cron.get_next(datetime)print(next_execution)
Moreover, Cronviter integrates seamlessly with other libraries and frameworks, enabling developers to embed complex scheduling logic within larger applications. For instance, integrating Cronviter with Django or Flask can streamline task scheduling within web applications. By leveraging the flexible API provided by Cronviter, developers can effortlessly incorporate cron-based scheduling into their existing workflows.
Additionally, Cronviter provides robust error handling and logging mechanisms. This ensures that any issues during the scheduling process can be promptly identified and addressed. The ability to customize logging levels and formats further enhances the monitoring and debugging capabilities.
Overall, Cronviter’s advanced features and customization options make it an indispensable tool for developers looking to master task scheduling in Python. Whether you need to manage simple periodic tasks or complex, multi-time-zone schedules, Cronviter offers the flexibility and power required to meet your needs.
Use Cases and Practical Applications
In the realm of task scheduling, Cronviter emerges as a powerful Python library, offering significant advantages for automating and managing cron expressions. Its versatility shines through in various real-world applications, enhancing efficiency and reliability across multiple domains.
One prominent use case for Cronviter is automating system maintenance tasks. Regular maintenance is crucial for the stability and performance of any system. With Cronviter, administrators can schedule tasks such as clearing temporary files, updating software, and performing backups at precise intervals. This ensures that maintenance routines are consistently executed, minimizing downtime and potential issues.
Another practical application is in scheduling periodic data processing jobs. In data-driven environments, timely processing of data is vital. Cronviter allows developers to set up cron expressions that trigger data extraction, transformation, and loading (ETL) processes at specific times. This automation not only streamlines workflows but also enhances data accuracy and timeliness, supporting better decision-making.
Cronviter also proves invaluable in managing timed notifications within web applications. User engagement can be significantly improved by sending timely alerts and reminders. Using Cronviter, developers can create schedules for sending notifications about upcoming events, subscription renewals, or personalized content updates. This contributes to a more dynamic and user-friendly experience.
The benefits of employing Cronviter in these contexts are evident. Its ability to handle complex scheduling requirements with ease reduces the need for manual intervention and helps maintain a seamless operational flow. Moreover, Cronviter’s integration with Python further simplifies the development process, making it accessible for both novice and experienced programmers.
In essence, Cronviter’s application in automating maintenance tasks, scheduling data processing jobs, and managing notifications illustrates its power and flexibility. By leveraging this robust library, organizations can achieve greater efficiency, reliability, and user satisfaction in their operations.
Conclusion and Further Resources
In this blog post, we have explored the significant benefits and functionalities that Cronviter offers as a Python library for handling cron expressions and task scheduling. We began by understanding the basics of cron expressions and their importance in automating repetitive tasks. Subsequently, we delved into the core features of Cronviter, highlighting its user-friendly API and robust capabilities for parsing, validating, and manipulating cron expressions.
Cronviter stands out due to its comprehensive error handling and ability to work seamlessly with various time zones, making it an indispensable tool for developers who require precision and reliability in scheduling tasks. The library’s versatility extends to both simple and complex scheduling needs, ensuring that users can efficiently manage their cron jobs with minimal effort.
Furthermore, Cronviter’s active community and extensive documentation provide a wealth of resources for both beginners and advanced users. Whether you are just starting out with cron expressions or looking to optimize your existing task scheduling workflows, Cronviter’s official documentation offers detailed guides and examples to help you get the most out of the library. Additionally, numerous tutorials and community forums are available to support your learning journey and address any challenges you may encounter.
For those interested in further exploring Cronviter, we recommend visiting the following resources:
Official Cronviter Documentation
Cronviter Questions on Stack Overflow
By leveraging these resources, you can deepen your understanding of Cronviter and enhance your ability to effectively schedule tasks using cron expressions. We hope this blog post has provided you with valuable insights and inspired you to incorporate Cronviter into your Python projects for more efficient and reliable task scheduling.
Technology
Exploring the Future of AI: Video Face Swap Technology and Its Uses

Artificial Intelligence (AI) has been making waves in various industries, and one of the most exciting developments is video face swap technology. This AI-powered tool allows users to seamlessly change a face in any video, creating captivating and sometimes humorous results. From entertainment to personal projects, face-swapping technology opens a world of possibilities. One leading platform to try this technology is the MioCreate Video Face Swap tool, which makes face-swapping in videos simple and accessible.
What is Video Face Swap AI Technology?
Video face swap AI technology uses advanced algorithms to map and superimpose one face onto another in a video. Unlike static image manipulation, this technology can handle complex video content, where the AI detects facial movements, expressions, and even lighting changes. The AI ensures that the swapped face moves naturally with the body, creating a seamless result.
The process has become much more user-friendly with tools like the MioCreate Video Face Swap Tool, which simplifies the face-swapping process for anyone, even those without technical skills. You simply upload a video and the face you want to replace, and the tool does the rest.
How Does Video Face Swap AI Technology Work?
Video face swap AI relies on deep learning models and neural networks to identify and replace facial features. Here’s a quick breakdown of how it works:
- Facial Recognition: The AI first analyzes the facial data from both the source video and the new face you want to use. It identifies key facial landmarks, such as the eyes, mouth, and nose.
- AI Training: The AI model has been trained using vast datasets of faces. This training allows it to recognize various facial expressions, head movements, and lighting changes, ensuring that the face swap is smooth and accurate.
- Face Superimposition: Once the AI recognizes and maps the face, it overlays the new face onto the original, taking care to match expressions and movements. This ensures that the new face looks natural throughout the video.
Steps to Use the MioCreate Video Face Swap Tool
The MioCreate Video Face Swap Tool makes it easy to swap faces in a video. Here’s how you can use it:
- Upload Your Video: Head to the MioCreate video face swap and upload the video where you want to swap a face.
- Upload the Face Image: You can choose from the preset faces and upload a picture with the face you want to use. Uploading a high-quality image can ensure a realistic face-swapped video.
- Process the Video: Click on “Swap Face Now” and then AI will start working its magic. It identifies and swaps the face with the selected one while maintaining all the original expressions and movements.
- Download and Share: After the process is complete, the output result will be a seamless face-swapped video ready to be saved and shared. You can download your video for free.
What Can Video Face Swap Technology Be Used For?
Video face swap AI is more than just a fun tool for creating entertaining videos. It can be used in various creative and practical applications:
- Social Media and Entertainment: Many people use video face swap technology to create humorous or creative content for social media platforms. Whether it’s swapping your face with a celebrity or a friend, the possibilities are endless.
- Creating Personalized AI Girlfriend Videos: Another emerging use of this technology is in personalized AI companion videos, such as those created with an AI Girlfriend. By using face swap technology, users can create interactive videos where the AI girlfriend’s face is customized to their preference.
- Marketing and Advertising: Brands can use face swap AI to personalize their advertisements. For instance, marketers can swap out a model’s face for a well-known influencer or celebrity to attract more attention to their campaigns.
- Education and Training: Educators can use this technology to make learning more engaging by swapping faces of historical figures or characters in educational videos. This can help students connect with the material in a fun and memorable way.
- Film and Video Production: For filmmakers, face swap AI offers a cost-effective way to alter scenes without expensive reshoots. By swapping actors’ faces, directors can modify scenes or create special effects with minimal effort.
Conclusion
Video face swap AI technology is revolutionizing how we create and interact with video content. With tools like the MioCreate Video Face Swap Tool, anyone can easily swap faces in videos for fun, marketing, or even more personal uses like creating an AI Girlfriend. Whether you’re using it to create viral social media posts or to enhance a film project, the possibilities with video face swap technology are truly endless. Dive into this fascinating technology and explore the creative opportunities it offers!
Technology
Understanding 127.0.0.1:62893: Meaning, Errors, and Solutions
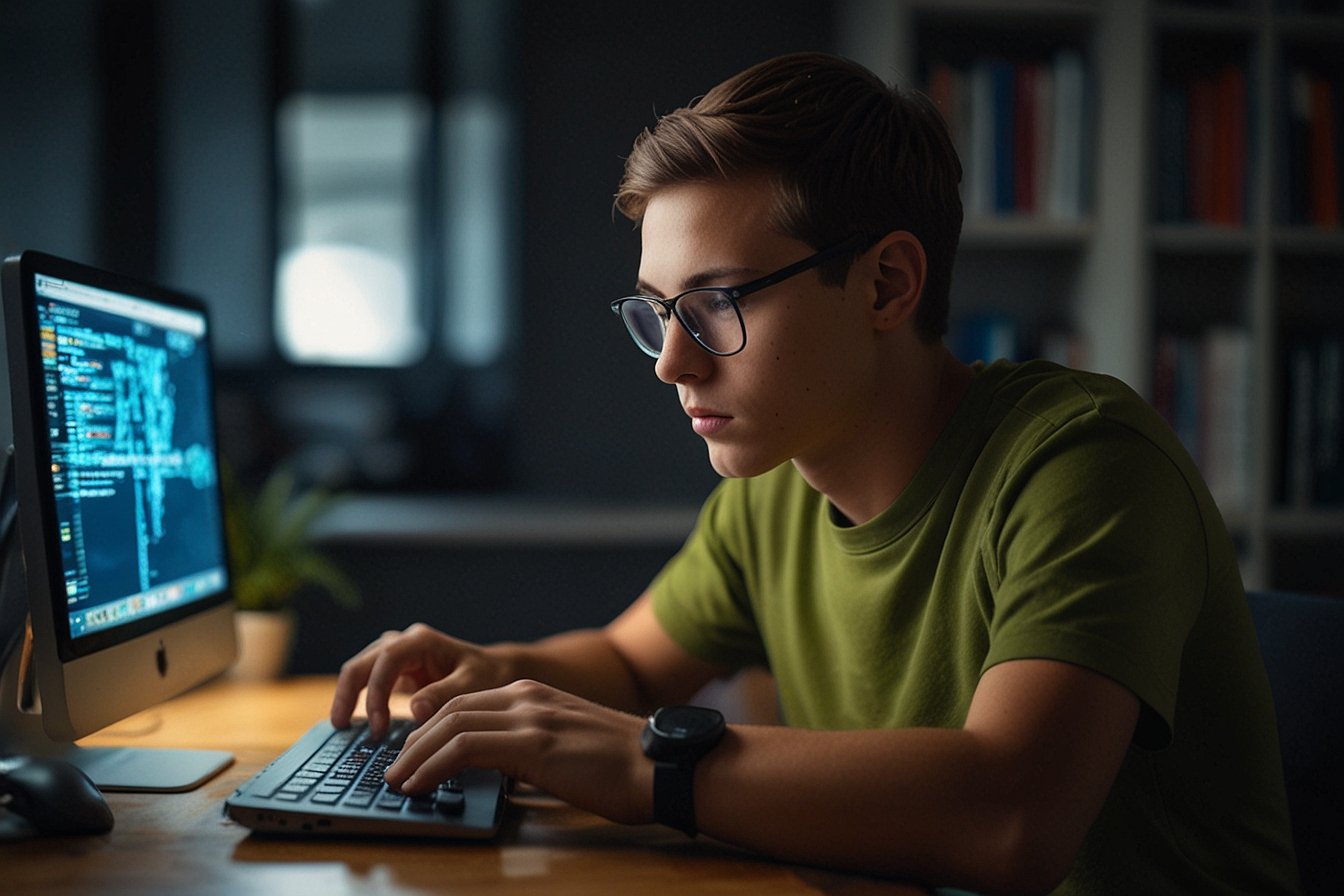
Encountering the code 127.0.0.1:62893 while working on your computer might seem perplexing, especially if it disrupts your development process with errors like “Disconnected from the target VM, address: 127.0.0.1:62893.” However, with a little insight, this seemingly complex code becomes easier to understand and manage. This article will explore what 127.0.0.1:62893 represents, how it functions, and how to resolve common issues associated with it.
What is 127.0.0.1:62893?
At its core, 127.0.0.1:62893 is a combination of an IP address and a port number:
- 127.0.0.1: This is the IP address for “localhost,” a special address used by your computer to refer to itself. It’s a loopback address, meaning that when your machine sends data to this address, it effectively sends the data back to itself without involving the external network. This is crucial for local testing and development.
- 62893: This is the port number, which acts as a specific channel for communication. While not as commonly known as ports like 80 (HTTP) or 443 (HTTPS), port 62893 is occasionally used by certain applications, such as Memcached, for internal data exchanges.
Why 127.0.0.1:62893 Matters
The address 127.0.0.1:62893 is more than just a technical curiosity. It serves several important purposes in computing and development:
- Development and Testing: Localhost is frequently used by developers to test applications on their machines without exposing them to the internet. This allows for a controlled and secure environment where bugs can be identified and fixed before the application is deployed.
- Understanding Networking: By experimenting with localhost and various port numbers, developers can deepen their understanding of how network communication works, including the intricacies of the TCP/IP protocol and client-server interactions.
- Security Considerations: Monitoring activity on ports like 62893 can help detect unauthorized access attempts. Keeping services on localhost rather than exposing them externally can also enhance security by limiting potential attack vectors.
- Efficient Debugging: When network-related issues arise, localhost provides a straightforward way to isolate and troubleshoot these problems, helping developers to quickly pinpoint and resolve errors.
- Creating Isolated Environments: Localhost creates a sandboxed environment where applications can run independently of other processes on the system, preventing conflicts and ensuring accurate testing results.
How 127.0.0.1:62893 Operates
The operation of 127.0.0.1:62893 can be broken down into a few simple steps:
- Initiating Communication: An application on your machine attempts to communicate with another process or service.
- Local Addressing: It uses “localhost” (127.0.0.1) as the destination, signaling that the interaction is meant to occur within the same device.
- Port Routing: The port number (62893) tells the operating system which specific service or application should handle the communication.
- Response and Interaction: The service on port 62893 responds accordingly, completing the data exchange loop entirely within the local environment.
Is it Safe to Expose Port 62893?
Exposing port 62893 to the public is generally not advisable. Here’s why:
- Potential Security Exploits: If a service like Memcached is running on this port with vulnerabilities, it could be targeted by attackers looking to gain unauthorized access to your system.
- Denial-of-Service (DoS) Risks: Publicly exposed ports can be targeted by DoS attacks, where an attacker floods the port with traffic, causing the system to crash or become unresponsive.
- Increased Risk of Unauthorized Access: If port 62893 is open to the internet, it becomes a potential entry point for hackers who might exploit weaknesses in the service running on this port.
Resolving the “Disconnected from the target VM, address: 127.0.0.1:62893” Error
This error message, often encountered during development, indicates that the debugger has lost its connection to the target virtual machine (VM). To fix this:
- Check the Service Status: Ensure the service or application tied to port 62893 is up and running. If it’s stopped, you’ll need to restart it.
- Verify Port Configuration: Double-check that the application is configured to use port 62893. If another service is using this port or if the port number has changed, update your settings accordingly.
- Firewall Adjustments: Make sure your firewall isn’t blocking local traffic on port 62893. You may need to add a rule to allow this specific port.
Steps to Fix the Error:
- Restart the Service: If the service associated with port 62893 is inactive, use system commands or tools like Task Manager (on Windows) to restart it.
- Change the Port Number: If there’s a port conflict, consider changing the port number in the application’s configuration. Ensure all related settings are updated to reflect this change.
- Adjust Firewall Settings: You can configure your firewall to allow traffic on port 62893:
- Windows:
- Navigate to Control Panel > System and Security > Windows Defender Firewall > Advanced Settings > Inbound Rules.
- Create a rule that permits inbound traffic on port 62893.
- Mac/Linux:
- Use terminal commands such as `iptables` to open port 62893. For example, the command might be `sudo iptables -A INPUT -p tcp –dport 62893 -j ACCEPT`.
- Check for Port Usage: Use tools like `netstat` on Windows or `lsof` on Unix/Linux to identify if another application is already using port 62893. This can help resolve port conflicts.
If the Problem Persists
If these solutions don’t resolve the issue:
- Consult Documentation: Check the official documentation for the software you’re using. It might offer specific troubleshooting advice for this type of error.
- Seek Professional Help: When in doubt, consider reaching out to an IT professional or service provider. Companies like Orage Technologies can provide expert assistance in resolving complex technical issues.
Orage Technologies: Your Partner in IT Solutions
Orage Technologies offers a range of services designed to address technical challenges like the one described here:
- Application Development: Their team of developers can quickly resolve errors such as “Disconnected from the target VM, address: 127.0.0.1:62893” and more, offering tailored solutions to meet your needs.
- Website Design and Development: Beyond fixing errors, Orage Technologies specializes in designing and developing secure, efficient websites.
- Cloud Solutions: Orage Technologies also provides cloud solutions for secure data storage and management, ensuring your systems remain efficient and accessible.
- Cybersecurity Services: If you encounter network-related errors, it’s crucial to assess your cybersecurity measures. Orage Technologies offers robust cybersecurity services to protect your infrastructure and data.
Conclusion
While 127.0.0.1:62893 might appear intimidating, it’s rooted in fundamental concepts of networking and development. This code combines an IP address (localhost) with a specific port number (62893) used for internal services, playing a crucial role in testing, debugging, and maintaining a secure development environment.
By understanding this code and learning how to troubleshoot related errors, you can improve your efficiency and maintain a secure working environment. Whether you’re a developer or an IT professional, mastering these concepts can significantly enhance your technical skill set and problem-solving capabilities.
Technology
Exploring Prince Narula’s Digital Journey: How PayPal Changed the Game
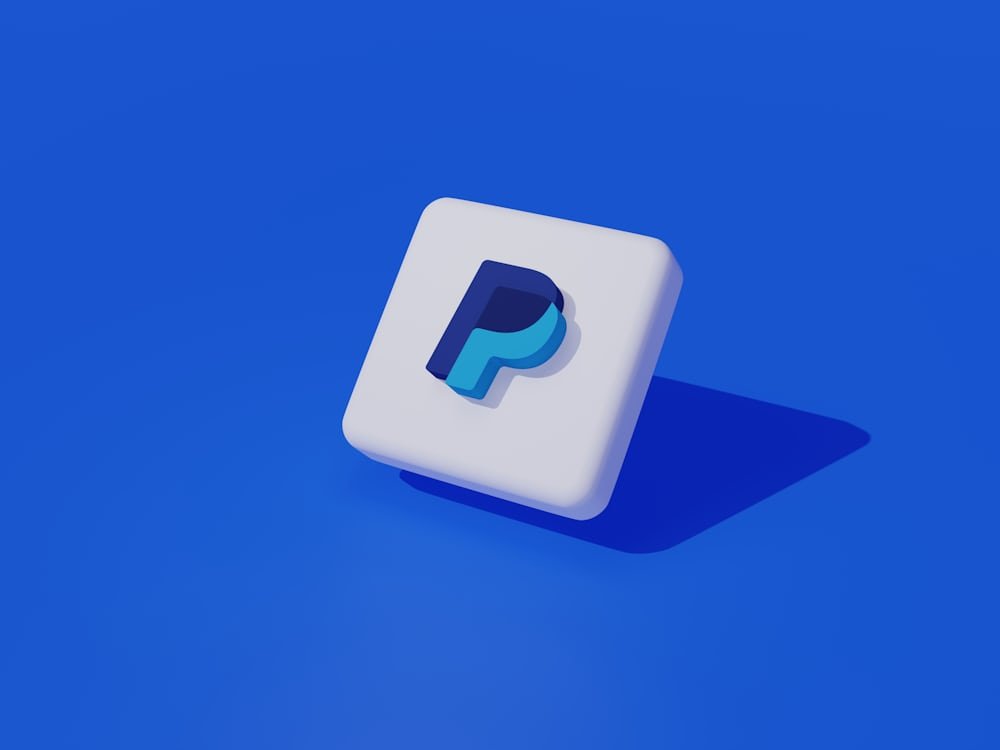
Introduction to Prince Narula and his success story
Prince Narula is a name that resonates with many, especially in the realm of Indian entertainment. From his early days as a contestant on reality shows to becoming a household name, Prince’s journey is nothing short of inspiring. But what truly sets him apart? It’s not just his talent or charisma; it’s how he embraced the digital world and leveraged it for success. In the context of “prince narula digital paypal”, his integration of digital tools has been pivotal.
In today’s fast-paced environment, having a strong digital presence isn’t just an option—it’s essential. This is where platforms like PayPal come into play, transforming lives and careers in remarkable ways. For Prince Narula, this was a game-changer that propelled him from struggling artist to celebrated star. His story reflects both resilience and ingenuity in navigating the challenges of the modern age. In this context, the role of “prince narula digital paypal” becomes pivotal.
Curious about how PayPal played such a pivotal role in shaping Prince’s career? Let’s dive deeper into his experiences and uncover valuable lessons we can all learn from them.
The importance of digital presence in today’s world
In today’s fast-paced world, having a digital presence is crucial. It’s no longer just an option; it’s a necessity. Everyone—from small businesses to celebrities—relies on online platforms to connect with their audience.
A strong digital footprint enhances visibility and credibility. People often search for products or personalities online before making any decisions. If you’re not present in that space, you risk being overlooked.
Moreover, social media has transformed how we engage with each other. It allows for direct interaction and feedback, creating a real sense of community around brands and individuals.
Digital presence also opens doors for opportunities that were once hard to reach. Networking has never been easier; collaborations can flourish through well-crafted content shared across various platforms. This is especially true in examples like "prince narula digital paypal.”
Whether you’re building your personal brand or promoting a business, understanding the importance of going digital is essential in this age of connectivity and information exchange.
Prince Narula’s early struggles and how PayPal helped him
Prince Narula faced significant challenges early in his career. Coming from a modest background, he grappled with financial constraints that limited his opportunities. His dreams of stardom felt distant and often unattainable.
During these tough times, digital platforms began to emerge as a lifeline. One such platform was PayPal, which transformed the way Prince managed his finances and transactions related to his burgeoning career. It provided him with an efficient means to receive payments for modeling gigs and appearances without facing traditional banking hurdles.
This newfound ease allowed him to focus on honing his craft instead of worrying about money flow issues. With every new project funded through seamless transactions via PayPal, Prince could invest more in self-promotion and branding efforts online. This shift marked a turning point in his journey toward success. This indeed showcases the notion of "prince narula digital paypal.”
How PayPal changed the game for Prince Narula’s career
PayPal played a transformative role in Prince Narula’s career. It provided him with the financial freedom he needed to invest in his passions. This was crucial during his early days when resources were limited.
With PayPal, managing transactions became seamless. He could quickly handle payments for various projects without hassle. This efficiency allowed him to focus on honing his skills and expanding his reach.
The digital platform also opened doors to collaborations that would have been challenging otherwise. Artists and brands began recognizing Prince’s potential, leading to partnerships that propelled him into the limelight.
In an industry where timing is everything, having instant access to funds made all the difference for Prince Narula. It enabled him not only to respond swiftly but also seize opportunities as they arose in a fast-paced environment like entertainment and social media. Clearly, “prince narula digital paypal” illustrates the vital impact of these digital tools.
The impact of social media on Prince Narula’s popularity
Social media has been a game changer for Prince Narula. Platforms like Instagram, Twitter, and Facebook have allowed him to connect directly with fans. This engagement fosters a sense of community around his brand.
His posts often showcase behind-the-scenes moments, giving followers an exclusive glimpse into his life. This transparency humanizes him, making fans feel more connected.
Moreover, social media serves as a powerful marketing tool for his projects. Whenever he stars in new shows or ventures into music, he can instantly share updates with his audience.
The real-time feedback from fans also helps shape his content and career decisions. As trends change quickly online, Prince adapts swiftly to maintain relevance.
Through creative content and engaging interactions, social media amplifies his reach beyond traditional celebrity platforms. It’s clear that this digital landscape plays a vital role in solidifying Prince Narula’s status as a pop culture icon. Thus, “prince narula digital paypal” becomes an integral aspect of understanding his digital journey.
Lessons we can learn from Prince Narula’s digital journey
Prince Narula’s digital journey offers valuable insights for anyone looking to thrive in the online world. His relentless determination is a testament to the power of perseverance. Embracing challenges head-on can lead to unexpected opportunities.
Social media has been pivotal in building his brand. Engaging authentically with followers helped him create a loyal fan base. This interaction not only fuels popularity but also fosters community.
Utilizing digital payment platforms like PayPal has streamlined his business transactions. It highlights how embracing technology can simplify processes and enhance efficiency in any venture.
Diversifying content across multiple platforms showcases adaptability. Exploring various formats keeps audiences engaged and broadens reach, ensuring that one remains relevant amidst changing trends. Along the lines of “prince narula digital paypal,” it showcases a holistic approach to digital success.
Conclusion: Embracing the power of digital platforms for success
As we look at Prince Narula’s journey, it’s clear that embracing digital platforms can be transformative. From his early struggles to becoming a household name, every step has been intertwined with his ability to adapt and leverage online tools like PayPal. This portrays the essence of "prince narula digital paypal.”
Digital presence is no longer optional; it’s essential for success. For aspiring entrepreneurs and influencers alike, the lessons from Prince’s career are invaluable. By utilizing technology effectively, one can transcend barriers and reach wider audiences.
Social media has reshaped how celebrities connect with their fans. It has given them a voice and a platform to share their stories authentically. Prince Narula capitalized on this trend, showcasing his personality while building an engaged community around him.
The world is changing rapidly, driven by digital innovation. Those who embrace these changes—not just in awareness but in action—will find doors opening where there seemed none before. The power of platforms like PayPal illustrates how financial tools can support creative endeavors while simplifying transactions.
Prince Narula’s story is not just about fame; it’s about resilience and innovation in the face of challenges. His journey serves as inspiration for anyone looking to navigate the evolving landscape of digital opportunities successfully. Success today hinges on understanding and leveraging these platforms wisely for personal growth or business advancement.